Checking Device Orientation in a Flutter App
// App0613.dartThe following images show what happens when you run the Dart code above. When the device is in portrait mode, you see two rows, with three boxes on each row. But when the device is in landscape mode, you see only one row, with six boxes.import 'package:flutter/material.dart';
import 'App06Main.dart';
Widget buildColumn(context) { if (MediaQuery.of(context).orientation == Orientation.landscape) { return _buildOneLargeRow(); } else { return _buildTwoSmallRows(); } }
Widget _buildOneLargeRow() { return Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ buildRoundedBox("Aardvark"), buildRoundedBox("Baboon"), buildRoundedBox("Unicorn"), buildRoundedBox("Eel"), buildRoundedBox("Emu"), buildRoundedBox("Platypus"), >, ), >, ); }
Widget _buildTwoSmallRows() { return Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ buildRoundedBox("Aardvark"), buildRoundedBox("Baboon"), buildRoundedBox("Unicorn"), >, ), SizedBox( height: 30.0, ), Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ buildRoundedBox("Eel"), buildRoundedBox("Emu"), buildRoundedBox("Platypus"), >, ), >, ); }
Here's the portrait option.
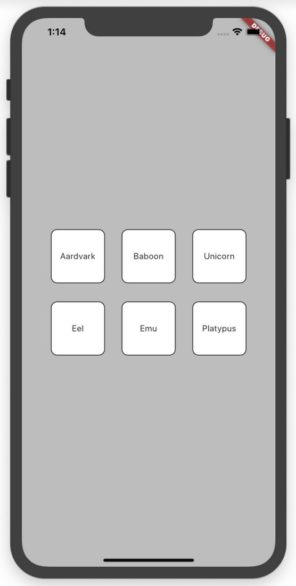
And the landscape mode.
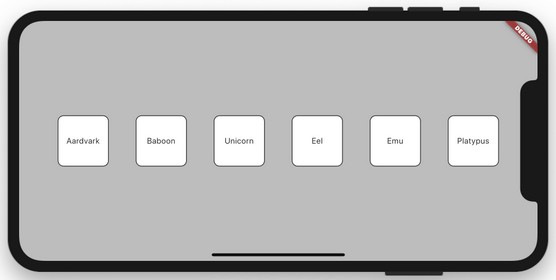
The difference comes about because of the if statement in the above Dart code.
if (MediaQuery.of(context).orientation == Orientation.landscape) { return _buildOneLargeRow(); } else { return _buildTwoSmallRows(); }Yes, the Dart programming language has an
if
statement. It works the same way that if statements work in other programming languages.
if (a certain condition is true) { Do this stuff; } otherwise { Do this other stuff; }In the name
MediaQuery
, the word Media
refers to the screen that runs your Flutter app. When you call MediaQuery.of(context)
, you get back a treasure trove of information about that screen, such as
orientation
: Whether the device is in portrait mode or landscape modeheight and size.width
: The number of dp units from top to bottom and across the device's screenlongestSide
andsize.shortestSide
: The larger and smaller screen size values, regardless of which is the height and which is the widthaspectRatio
: The screen's width divided by its heightdevicePixelRatio
: The number of physical pixels for each dp unitpadding
,viewInsets
, andviewPadding
: The parts of the display that aren't available to the Flutter app developer, such as the parts covered up by the phone's notch or (at times) the soft keyboardalwaysUse24HourFormat
: The device's time display settingplatformBrightness
: The device's current brightness setting- . . . and many more
orientation
property. In that case, you can replace the condition in the code above with something like the following:
if (MediaQuery.of(context).size.width >= 500.0) { return _buildOneLargeRow(); } else { return _buildTwoSmallRows(); }Notice the word
context
in the code MediaQuery.of(context)
. In order to query media, Flutter has to know the context in which the app is running. That's why, _MyHomePage
class's build
method has a BuildContext
context parameter.Want to learn more tricks? Avoid these ten mistakes when programming your Flutter app.