How Old Are You?
import 'package:flutter/material.dart';The images below show the Flutter app in its two possible states.void main() => runApp(App0701());
class App0701 extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } }
class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); }
<strong>const _youAre = 'You are'; const _compatible = 'compatible with\nDoris D. Developer.';</strong>
class _MyHomePageState extends State { <strong> bool _ageSwitchValue = false; String _messageToUser = "$_youAre NOT $_compatible"; </strong> /// State
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Are you compatible with Doris?"), ), body: Padding( padding: const EdgeInsets.all(8.0), child: Column( children: [ _buildAgeSwitch(), _buildResultArea(), >, ), ), ); }
/// Build
Widget _buildAgeSwitch() { return Row( children: [ Text("Are you 18 or older?"), <strong> Switch( value: _ageSwitchValue, onChanged: _updateAgeSwitch,</strong> ), >, ); }
Widget _buildResultArea() { return Text(_messageToUser, textAlign: TextAlign.center); }
/// Actions
<strong> void _updateAgeSwitch(bool newValue) { setState(() { _ageSwitchValue = newValue; _messageToUser = _youAre + (_ageSwitchValue ? " " : " NOT ") + _compatible; }); } }</strong>
Here’s one state.
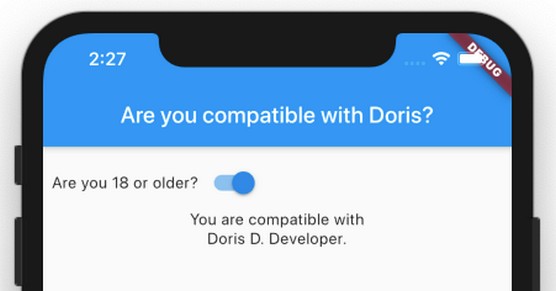
And here’s the other.

What you find here are practice apps. They're bite-size samples of an example app called Doris's big, beautiful dating app. But even "bite-size" programs can be long and complicated. Sometimes, a few of the rules are bent and some good programming practices are ignored to make the listings compatible with one another. But, don't worry. Each listing works correctly, and each listing illustrates useful Flutter development concepts.
You can find the entire dating app at this Flutter code resource.The Flutter code above has an onChanged
parameter. The onChanged
parameter's value is a function; namely, the _updateAgeSwitch
function. When the user flips the switch, that flip triggers the switch's onChanged
event, causing the Flutter framework to call the _updateAgeSwitch
function.
Note that the _updateAgeSwitch
function in the code above isn't a VoidCallback
. A VoidCallback
function takes no parameters, but the _updateAgeSwitch
function has a parameter. The parameter's name is newValue
:
void _updateAgeSwitch(bool newValue)When the Flutter framework calls _updateAgeSwitch, the framework passes the Switch widget's new position (off or on) to the newValue parameter. Because the type of newValue is bool, newValue is either false or true. It's false when the switch is off and true when the switch is on.
If _updateAgeSwitch
isn't a VoidCallback
, what is it? (That was a rhetorical question, so let’s answer it for you. . . .) The _updateAgeSwitch
function is of type ValueChanged<bool>
. A ValueChanged
function takes one parameter and returns void. The function's parameter can be of any type, but a ValueChanged<bool>
function's parameter must be of type bool
. In the same way, a ValueChanged<double>
function's parameter must be of type double
. And so on.
Make no mistake about it: Even though the term ValueChanged
doesn't have the word Callback
in it, the _updateAgeSwitch
function is a callback. When the user flips the Switch
widget, the Flutter framework calls your code back. Yes, the _updateAgeSwitch
function is a callback. It's just not a VoidCallback
.
With many controls, nothing much happens if you don't change the control's value and call setState. For a few laughs, let’s try commenting out the setState call in the body of the _updateAgeSwitch function listed above:
void _updateAgeSwitch(bool newValue) { <strong> // setState(() {</strong> _ageSwitchValue = newValue; _messageToUser = _youAre + (_ageSwitchValue ? " " : " NOT ") + _compatible; // }); }Next, let’s uncomment the setState call and comment out the assignment statements:
void _updateAgeSwitch(bool newValue) { setState(() { <strong>// _ageSwitchValue = newValue; // _messageToUser = // _youAre + (_ageSwitchValue ? " " : " NOT ") + _compatible;</strong> }); }In both cases, the program was restarted and then the switch was tapped. Not only did the
_messageToUser
refuse to change, but the switch didn't even budge. That settles it! The look of the switch is completely dependent on the _ageSwitchValue
variable and the call to setState
. If you don't assign anything to _ageSwitchValue
or you don't call setState
, the switch is completely unresponsive.Want to learn more? Check out our Flutter Cheat Sheet.