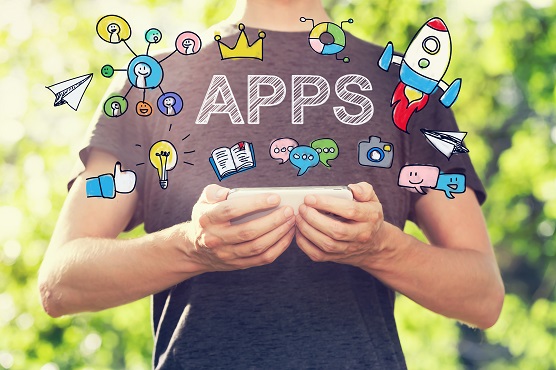
Java and Kotlin for Android app development
James Gosling from Sun Microsystems created the Java programming language in the mid-1990s. (Sun Microsystems has since been bought out by Oracle.) Java's meteoric rise in use came from the elegance of the language and the well-conceived platform architecture. After a brief blaze of glory with applets and the web, Java settled into being a solid, general-purpose language with special strength in servers and middleware.In the meantime, Java was quietly seeping into embedded processors.
An embedded processor is a computer chip that's hidden from the user as part of some special-purpose device. The chips in today's cars are embedded processors, and the silicon that powers your photocopier at work is an embedded processor. Pretty soon, the flowerpots on your windowsill will probably have embedded processors. By 2002, Sun Microsystems was developing Java ME (Mobile Edition) for creating MIDlets based on the Mobile Information Device Profile (MIDP) to run on mobile phones in a manner similar to applets on a web page. Java became a major technology in Blu-ray disc players, parking meters, teller machines, and other devices. So, the decision to make Java the primary development language for Android apps was no big surprise.
The trouble was, not everyone agreed about the fine points of Java's licensing terms. The Java language isn't quite the same animal as the Java software libraries, which in turn aren't the same as the Java Virtual Machine (the software that enables the running of Java programs).So, in marrying Java to Android, the founders of Android added an extra puzzle piece — the Dalvik Virtual Machine. And instead of using the official Sun/Oracle Java libraries, Android used Harmony — an open-source Java implementation from the Apache Software Foundation. Several years and many lawsuits later, Google and Oracle were still at odds over the use of Java in Android phones.
Programmers always use one kind of software to develop other kinds of software. For several years, programmers had developed Android apps using software named Eclipse. In the meantime, a competing product named IntelliJ IDEA was growing in popularity. A group of engineers from Google and IntelliJ IDEA's parent company (JetBrains) cooperated to create a very lean version of IntelliJ IDEA.
Simply put, they removed the features of IntelliJ IDEA that Android developers would never use. From this stripped-down version of IntelliJ IDEA, they created a customized product especially for Android programmers. At its annual developer conference in 2013, Google introduced Android Studio — the shiny new tool to help Android developers be more productive.
Also at the aforementioned 2013 developer conference, Google began the process of replacing Dalvik with a new virtual machine named Android Runtime, or ART. Programs ran faster under ART, plus they consumed less memory and less power, and they were easier to debug. The transition from Dalvik to ART was a first step in the separation of Android from Oracle's proprietary Java technologies.
While Android matured, new programming languages were making improvements on many of Java's long-standing features. Apple was developing Swift to replace the aging Objective-C language. With Swift and other such languages, developers had a natural way of controlling how values may change during the run of a program.
Developers could easily extend existing functionality and create code to tame null values — values that expert Tony Hoare had dubbed a "billion-dollar mistake." In newer languages, programmers used built-in features to write code in a functional style (a coding technique that expresses computational goals in the form of math functions).
JetBrains was developing one of these new languages. The language's name — Kotlin — came from the name of an island near St. Petersburg in Russia. Kotlin borrowed many of Java's features and improved on them in significant ways. At its annual developer conference in 2017, Google announced support for creating Android programs using Kotlin.
A year later, 35 percent of all Android programmers were using Kotlin. In 2019, Google officially dubbed Kotlin as the preferred language for Android app development.
Kotlin is fully compatible with Java. So, when you create an Android app, you can borrow pieces of programs written in Java and meld them seamlessly with your Kotlin code. Kotlin also integrates with JavaScript, so developers can write Kotlin programs that drive web pages.
Behind the scenes, Kotlin plays nicely with Node.js — a widely-used platform that runs on servers around the world. You can even translate Kotlin into native code — code that runs on Windows, macOS, Linux, and other operating systems.
Kotlin is deeply entrenched in the Android ecosystem and in other systems as well. If you already have some Kotlin programming experience, great! If not, there are plenty of resources to help you broaden your horizons into Kotlin programming.XML for Android app development
If you find View Source among your web browser's options, you see a bunch of Hypertext Markup Language (HTML) tags that represent the coding for a web page. A tag is some text enclosed in angle brackets. The tag describes something about its neighboring content.For example, to create boldface type on a web page, a web designer writes
Look at this!
The angle-bracketed b
tags turn boldface type on and off.
The M in HTML stands for Markup — a general term describing any extra text that annotates a document's content. When you annotate a document's content, you embed information about the document's content into the document itself. So, for example, in the line of code in the previous paragraph, the content is Look at this!
The markup (information about the content) consists of the tags <b>
and </b>
.
The HTML standard is an outgrowth of SGML (Standard Generalized Markup Language). SGML is an all-things-to-all-people technology for marking up documents for use by all kinds of computers running all kinds of software and sold by all kinds of vendors.
In the mid-1990s, a working group of the World Wide Web Consortium (W3C) began developing XML — the eXtensible Markup Language. The working group's goal was to create a subset of SGML for use in transmitting data over the Internet. The group succeeded. Today, XML is a well-established standard for encoding information of all kinds. Java is good for describing step-by-step instructions, and XML is good for describing the way things are (or should be). A Java program says, “Do this and then do that.” In contrast, an XML document says, “It's this way, and it's that way.” Android uses XML for two purposes:
- To describe an app's data. An app's XML documents describe the look of the app's screens, the translations of the app into one or more languages, and other kinds of data.
- To describe the app itself. Each Android app comes with an xml file. This XML document describes features of the app. The operating system uses the
AndroidManifest.xml
document's contents to manage the running of the app.
AndroidManifest.xml
file contains the app’s name and the name of the file containing the app’s icon. The XML file also lists the names of the app’s screens and tells the system what kinds of work each screen can perform.For more information about the AndroidManifest.xml
file and about the use of XML to describe an app's data.
Concerning XML, there's bad news and good news. The bad news is, XML isn't always easy to compose. The good news is, automated software tools compose most of the world's XML code. The software on an Android programmer’s development computer composes much of an app's XML code. You often tweak the XML code, read part of the code for info from its source, make minor changes, and compose brief additions. But you hardly ever create XML documents from scratch.
When you create an Android app, you deal with at least two “computers.” Your development computer is the computer that you use for creating Android code. (In most cases, your development computer is a desktop or laptop computer — a PC, Mac, or Linux computer.) The other computer is something that most people don't even call a “computer.” It's the Android device that will eventually be running your app. This device is a smartphone, tablet, watch, or some other cool gadget.
Linux for Android app development
An operating system is a big program that manages the overall running of a computer or device. Most operating systems are built in layers. An operating system's outer layers, such as the applications and the environment in which the user interacts with the applications, are usually right up there in the user's face.For example, both Windows and macOS have standard desktops (a paradigm for the interface the user sees, sort of like the physical desktop you use at work). From the desktop, the user launches programs, manages windows, and so on. When you’re working with Android, the desktop is represented by the Android Application Framework.
An operating system's inner layers are (for the most part) invisible to the user. While the user plays Solitaire, the operating system juggles processes, manages files, keeps an eye on security, and generally does the kinds of things that the user shouldn't micromanage. The image below shows the Android version of these inner layers as Libraries and the Android Runtime.
At the very deepest level of an operating system is the system's kernel. The kernel runs directly on the processor's hardware and does the low-level work required to make the processor run. In a truly layered system, higher layers accomplish work by making calls to lower layers. So an app with a specific hardware request sends the request (directly or indirectly) through the kernel.
The best-known, best-loved general-purpose operating systems are Microsoft Windows, Apple macOS (which is built on top of UNIX), and Linux (from various vendors). Windows and macOS are the properties of their respective companies. But Linux is open source. That's one of the reasons why the creators of Android based their platform on the Linux kernel. Openness is a good thing!
Rules concerning the use of open-source software come in many shapes and sizes. For example, there's the GNU General Public License (GPL), the Apache License, the GNU Lesser General Public License (LGPL), and others. When considering the use of other people's open-source software, be careful to check the software's licensing terms. “Open source” doesn't necessarily mean “do anything at all for free with this software.”
Here's a ten-cent tour of the Android operating system:- first, you have the applications — the web browser, the contacts list, the games, the dialer, the camera app, and other goodies. Developers and users interact with this layer. Developers write code to run on this layer, and users see the screens created by apps in this layer.
- Below the applications layer lies the Application Programming Interface (API) layer. Your Android programs make requests to pieces of code in this API layer.As an Android developer, you almost never deal with layers below the API layer. But just this once, you can take a quick peek at those three layers. Here goes . . .
- Android's middle layer has two parts: a bunch of code written in the C and C++ programming languages; and the Android Runtime (ART). The Android Runtime is a workhorse that runs all your Kotlin code.
- The Hardware Abstraction Layer (HAL) is a kind of universal translator. Imagine having guests from many foreign countries and speaking none of their languages. You can learn all their languages, but hiring several translators is easier. That's how HAL works. The Android Runtime runs on many different kinds of hardware, but ART isn't tailored for any particular kind of hardware. Instead, ART hires a HAL for each kind of hardware. Pretty clever!
- At the bottom is the Linux kernel, managing various parts of a device's hardware. The kernel includes a Binder, which handles all communication among running processes. When your app asks, “Can any software on this phone tell me the current temperature in Cleveland, Ohio?” the request for information goes through the kernel's Binder.
cd
to change to a directory, ls
to list a directory's files and subdirectories, rm
to delete files, and many others.The Google Play Store has plenty of free terminal apps. A terminal app's interface is a plain text screen in which you type Linux shell commands. And with one of Android's developer tools, the Android Debug Bridge, you can issue shell commands to an Android device through your development computer. If you like getting your virtual hands dirty, the Linux shell is for you.
Want to learn more? Check out our Android App Development Cheat Sheet.