Using pseudocode to make an object move
Each programming language uses its own special command to move an object, and you can write different pseudocode expressions to represent the process of moving an object onscreen. Themove <em>number</em>
(measured in number of pixels) pseudocode command represents moving an object some distance in the direction in which the object is pointing. An example is move 5
or move 15
, with the larger number representing a larger distance moved.Another way to make an object move is to change its x-coordinate or its y-coordinate.
changex <em>number</em> changey <em>number</em>Changing the x-coordinate forever creates horizontal motion. Changing the y-coordinate forever creates vertical motion.
To make an object move constantly, place a motion command inside a forever
command:
forever [move <em>number</em>> forever [changex <em>number</em>> forever [changey <em>number</em>>
Move forever commands are useful for making games and models. For example, coding several cars to move forever in the horizontal direction allows your coder to create traffic for a Crossy Road-style game.
Using Scratch to make an object move
To make an object move in Scratch, set the direction of the object using two commands: thepoint in direction <em>angle</em>
command in the Motion category, and the move <em>number</em> steps
in the Operators category. The range of point in direction
is 0 to 360 degrees. For the move
command, use any number; negative numbers move the object backwards. The larger the number, the bigger the move. Note that move makes
the sprite object move in a straight line, not spin in place.
point in direction angle
command and the move number steps number
command to move an object in Scratch.To create horizontal motion, use change x by <em>number</em>
using any number; negative numbers move the object to the left and positive numbers move the object to the right.
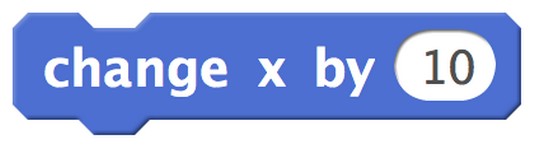
change x by number
command to move an object horizontally in Scratch.To create vertical motion, use change y by <em>number</em>
using any number; positive numbers move the object up and negative numbers move the object down.
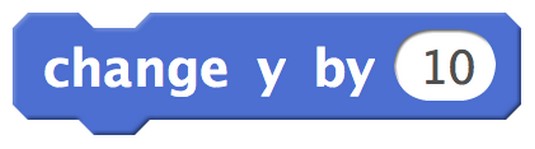
change y by number
command to move an object vertically in Scratch.To make an object move constantly, place any motion command inside a forever
command. This image shows the shows the move <em>number</em> steps
inside forever
.

move
command inside the forever
command to create constant motion in Scratch.Scratch doesn't have a wrapping feature on its stage. When a moving object reaches the edge of the screen, it stops. You can counteract this by making an object bounce off the side of the screen. To do so, add an if on edge, bounce
command.

if on edge, bounce
command to make an object bounce off the edge of the stage.Another way you can keep an object moving at the edge of the stage in Scratch is to create your own code for wrapping. You can make an object leave one edge of the stage, and then appear at the opposite edge of the stage. The image below shows code for an object that moves right and when it reaches the right side of the stage instantly repositions itself on the left side of the stage. You can find the inequality operators in the Operators category.

Using JavaScript to make an object move
To make an object move in JavaScript, you code the starting position of the object and then make the position change a small quantity during a time interval.The following code moves a mouse object left to right, across the screen in the Code.org App Lab, using JavaScript. The mouse x-coordinate begins at 0. Its y-coordinate stays constant at 150.
image("character", "mouse.png"); var xpos = 0; timedLoop(250, function () { xpos = xpos + 1; setPosition("character", xpos, 150, 100, 100); });Here's what each line of code does in this program:
- Line 1 identifies the object you want to move. For example,
image("character", "mouse.png");
- Line 2 creates a variable for x-position of the object (
xpos
) if you want to make the object move horizontally. Alternatively, create a variable for the y-position of the object (ypos
) if you want the object to move vertically. Initialize the variable by setting it equal to the starting value for the object. For example, varxpos = 0;
. - Line 3 creates a timed loop to indicate how often the object will move. In a timed loop, 1000 equals 1 second. A time loop set at 250 executes one time every quarter-second.
- Line 4 increments the position variable inside the timed loop. This causes the position of the object to change a little each time the loop executes. For example,
xpos = xpos + 1;
. - Line 5 uses a
setPosition
command inside the timed loop to set the object x-coordinate toxpos
.