You may not have explored any Swift syntax yet, but you can still experiment with it. Here, you learn how to test the results of a line of code in a playground, and then how to check the syntax of your code within a playground.
Testing a line of code
The results of any code you type into the playground can be seen more or less instantly. To illustrate, begin with the playground shown here.
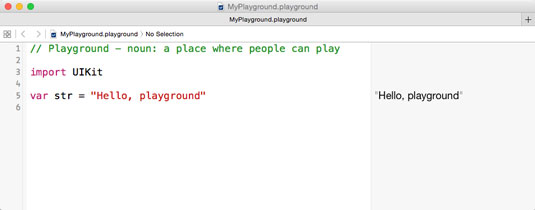
Then change the value of str with these steps:
Add a line to change the value of str to “Another String” as shown.
Look at the sidebar to see the new value.
The original value of str, “Hello, playground,” is now changed to “Another String.”
This is the pattern for using playgrounds in Swift: Just type something in the playground and see the new result in the sidebar. The result in the sidebar may or may not be what you were looking for, but you can still respond accordingly, as follows:
If the value is what you expect, you’re done (and you’re successful!).
If nothing changes in the sidebar (that is, if no value appears or if the original value there remains unchanged), check for a syntax error. If the playground can’t interpret your code, it’s not going to execute it.
If you see the wrong result, check your code. A flaw in logic may have given you the wrong answer.
Depending on your Mac’s processor speed, the other apps or processes you have running, and the complexity of your code, there may be a delay while Swift parses and then executes the code. At least in early versions of playgrounds in Xcode 6, some developers reported that it was sometimes necessary to give the playground a little nudge. Changing your code a little (such as deleting a word or two and then adding it back in) may cause the parser to be reinvigorated.
Checking syntax
One of the best things to do with a playground is to check Swift syntax quickly. Here’s an example of that kind of use. (Note that it involves a deliberate typo.)
Swift is type‐safe, which means that it requires you to explicitly do your own type conversions. How do you convert an integer to a string? This is a simple task, but if you’re familiar with several languages, it’s easy to forget which language uses which syntax. In such cases, a playground can be very useful: You just open a playground and try a variety of syntax approaches until you get the answer you want. The following steps show you how:
Create a playground.
Open the Assistant (the two overlapping circles at the top right of the window shown here).
The listing shows the completed code you create in your playground.
// Playground - noun: a place where people can play import UIKit var str = String(1) var str2: Int = 1
This opens a second pane in the playground, just as it does in Xcode editing windows. If you don’t see the overlapping circles, choose View→Show Toolbar.
In the main pane (the leftmost pane), type in your first guess at the code.
After the import line and the var line, enter
str = (String)1
If the syntax is incorrect, the playground shows you the errors. Note that in addition to the errors shown on the right, Xcode offers a Fix‐It solution. If your syntax is incorrect, the suggested Fix‐It may be wrong (as it is in this case). However, the errors displayed in Console Output at the right of the window show you the actual error: It’s in the conversion to String:
str = (String)1
Type in the correct code:
str = String(1)
Check the right-hand sidebar to see the result.
The value shown, “1,” is correct. This is the correct number, and the quotes correctly indicate that the value is a string, as shown.
Verify the result by adding another line.
Here make the conversion from string to integer by adding
var str2: Int = 1
Check the result in the sidebar, as shown.
Note that this new value is an Int and not a String, which you can tell by the absence of quotes.