You can modify the content of a list as needed with Python. Modifying a list means to change a particular entry, add a new entry, or remove an existing entry. To perform these tasks, you must sometimes read an entry. The concept of modification is found within the acronym CRUD, which stands for Create, Read, Update, and Delete. Here are the list functions associated with CRUD:
append(): Adds a new entry to the end of the list.
clear(): Removes all entries from the list.
copy(): Creates a copy of the current list and places it in a new list.
extend(): Adds items from an existing list and into the current list.
insert(): Adds a new entry to the position specified in the list.
pop(): Removes an entry from the end of the list.
remove(): Removes an entry from the specified position in the list.
The following steps show how to perform modification tasks with lists.
Open a Python Shell window.
You see the familiar Python prompt.
Type List1 = [] and press Enter.
Python creates a list named List1 for you.
Notice that the square brackets are empty. List1 doesn’t contain any entries. You can create empty lists that you fill with information later. In fact, this is precisely how many lists start because you usually don’t know what information they will contain until the user interacts with the list.
Type len(List1) and press Enter.
The len() function outputs 0. When creating an application, you can check for an empty list using the len() function. If a list is empty, you can’t perform tasks such as removing elements from it because there is nothing to remove.
Type List1.append(1) and press Enter.

Check for empty lists as needed in your application.
Type len(List1) and press Enter.
The len() function now reports a length of 1.
Type List1[0] and press Enter.
![Type List1[0] and press Enter.](https://cdn.prod.website-files.com/6634a8f8dd9b2a63c9e6be83/66ccb75e63771094d3e99164_445030.image1.jpeg)
You see the value stored in element 0 of List1.
Type List1.insert(0, 2) and press Enter.
The insert() function requires two arguments. The first argument is the index of the insertion, which is element 0 in this case. The second argument is the object you want inserted at that point, which is 2 in this case.
Type List1 and press Enter.
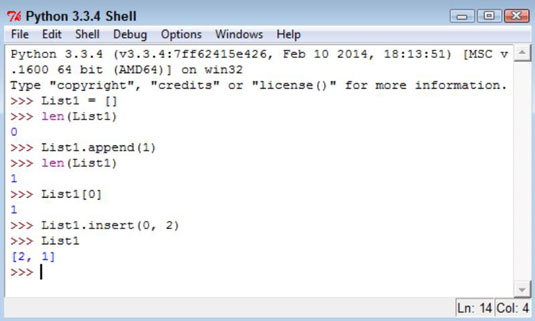
Python has added another element to List1. However, using the insert() function lets you add the new element before the first element.
Type List2 = List1.copy() and press Enter.
The new list, List2, is a precise copy of List1. Copying is often used to create a temporary version of an existing list so that a user can make temporary modifications to it rather than to the original list. When the user is done, the application can either delete the temporary list or copy it to the original list.
Type List1.extend(List2) and press Enter.
Python copies all the elements in List2 to the end of List1. Extending is commonly used to consolidate two lists.
Type List1 and press Enter.

You see that the copy and extend processes have worked. List1 now contains the values 2, 1, 2, and 1.
Type List1.pop() and press Enter.
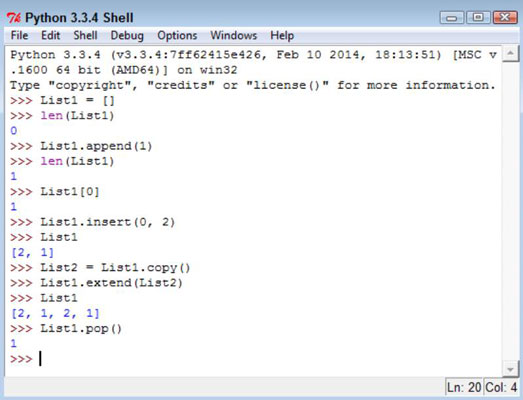
Python displays a value of 1. The 1 was stored at the end of the list, and pop() always removes values from the end.
Type List1.remove(1) and press Enter.
This time, Python removes the item at element 1. Unlike the pop() function, the remove() function doesn’t display the value of the item it removed.
Type List1.clear() and press Enter.
Using clear() means that the list shouldn’t contain any elements now.
Type len(List1) and press Enter.
You see that the output is 0. List1 is definitely empty. At this point, you’ve tried all the modification methods that Python provides for lists. Work with List1 some more using these various functions until you feel comfortable making changes to the list.
Close the Python Shell window.
Congratulations on a job well done!