JavaScript code writers need to have a form grasp on geolocation. The Geolocation API gives programs access to the web browser’s geolocation functionality, which can tell the program the device’s location on Earth. The Geolocation API is among the most well-supported HTML5 APIs and is implemented in about 90 percent of desktop and mobile browsers, including all of the big ones, except for Opera Mini.
What does geolocation do?
The Geolocation API describes how JavaScript can interact with the
navigator.geolocation object in order to get data about a device’s current position, including
Latitude: The latitude in decimal degrees
Longitude: The longitude in decimal degrees
Altitude: The altitude in meters
Heading: The direction the device is traveling
Speed: The velocity of the device in meters per second
Accuracy: The accuracy of the latitude and longitude, measured in meters
By obtaining some or all of this data, a JavaScript application running in a web browser can place a user on a map, query sources such as Google Maps for landmarks or restaurants local to the user, and much more.
How does geolocation work?
When JavaScript initiates a request for the devices position, through the Geolocation object, a number of steps take place, prior to the position information being returned.
The first thing to happen is that the browser needs to make sure that the user has given permission for the particular web app to access the device’s geolocation information. Different browsers prompt the user for permission in different ways, but it’s typically done through some sort of popup or notification.
The Chrome browser displays a geolocation icon and a message below the address bar when a website requests access to geolocation data.
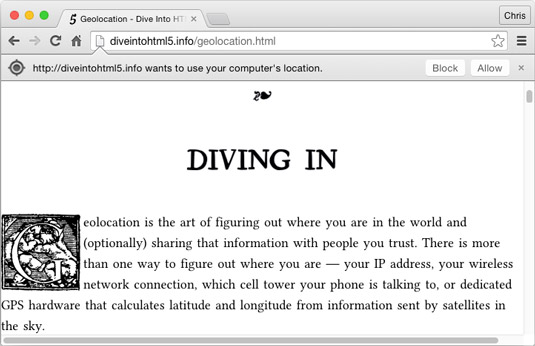
After you give a website access to your geolocation data, the browser tries to find you. It does this through a number of different means, starting with the most accurate and proceeding through to less accurate ways.
If the program indicates that high accuracy is required, geolocation will spend a longer time trying to access highly accurate GPS information. Otherwise, the browser will attempt to balance speed with accuracy to obtain the most accurate results from any of the following sources, when available:
GPS satellite positioning
Your wireless network connection
The cell tower your phone or mobile device is connected to
The IP address of your device or computer
How do you use geolocation?
The key to using geolocation is the navigator.geolocation object’s getCurrentPosition method. The getCurrentPosition method can take three arguments:
success: A callback function that’s passed a Position object when geolocation is successful
error: An optional callback function that’s passed the PositionError object when geolocation fails
options: An optional PositionOptions object that can be used to control several aspects of how the geolocation lookup is performed
The Position object that’s returned by the getCurrentPosition method contains two properties:
Position.coords: Contains a Coordinates object that describes the location
Position.timestamp: The time when the location was retrieved
This example shows how you can use the getCurrentPosition method to get the Position object and loop through the return values in Position.coords.
<html> <head> <title>The Position object</title> <script> var gps = navigator.geolocation.getCurrentPosition( function (position) { for (key in position.coords) { document.write(key+’: ‘+ position.coords[key]); document.write (‘<br>‘); } }); </script> </head> <body> </body> </html>
If the device you run this code on supports geolocation and the browser can determine your location, the results of running this script should resemble this.
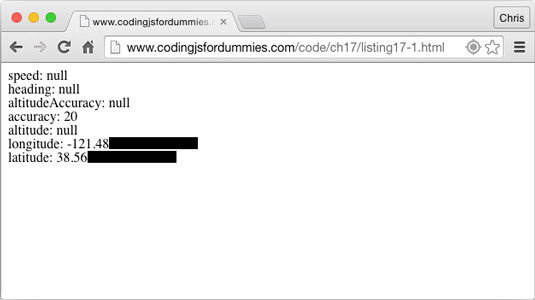
Notice that several of the properties of the Coordinates object are all null. This is because it was run using a desktop computer that doesn’t have the ability to get some of these coordinates. The result of running the same script in a mobile browser on a smartphone:

Notice that the mobile browser displays figures for altitude, but heading and speed are still null because the device was stationary at the time when the page was loaded.