However, if you learn a wide variety of tools, you'll understand how libraries and frameworks are constantly improving on what's been done before, and you'll gain an appreciation for why change is so important in the JavaScript world.
Finding and choosing Node.js packages
The npm Registry contains well over 1 million packages, all of which are open source. With so much software and so many libraries available to JavaScript developers, you can usually find a library or tool that already exists to handle just about any day-to-day task, and many less common jobs.
Searching for packages
To search for a package in the npm Registry, you can enter a keyword or package name into the search box that’s at the top of every page in the Registry.
For example, if you’re looking for a unit testing tool, enter “unit testing” into the npm Registry search bar and you’ll get back about 1,500 different packages that related to unit testing.
Understanding the search rank criteria
With so many packages available that do similar things, how do you choose one? Three important criteria to consider when evaluating Node.js packages are popularity, quality, and maintenance.
In the npm Registry search results pages, each package is ranked according to the three criteria and a small chart to the right of each package description shows a green line labeled “p” (for popularity), a purple line labeled “q” (for quality), and a red line labeled “m” (for maintenance), as shown in the following figure.
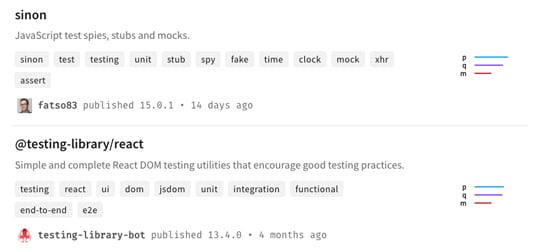
npm Registry results page
- Popularity is measured by the number of times a package has been downloaded. The idea is that if more people download a package, that’s a good indication that the package is useful. In the example results when searching for “unit testing”, the packages range in popularity from Sinon.JS, with 3 million downloads per week, to packages that may get 100 downloads in a good week.
- Quality of package is based on characteristics of the package that generally corollate with how “good” a package is. These factors include whether the package has a README file, whether it includes tests, whether the dependencies it uses are up-to-date, whether it has its own website, and how complex the code is.
- Maintenance determines how much attention the developers of the package give to it. It’s determined by looking at how frequently and how recently updates to the package have been committed. With dependencies constantly updating and ever-evolving security threats, it’s critical that packages get regular attention. A package that isn’t maintained will quickly become broken, useless, or even dangerous to use.
On the left of the Registry’s search results are four radio buttons for choosing the sort order of your search results, as shown in the following figure:
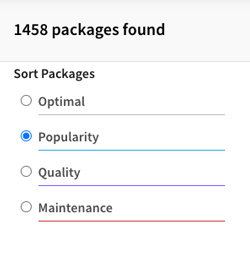
Sort order options on npm Registry results page
In addition to the three search rank criteria (popularity, quality, and maintenance), you can also choose to sort by a criteria called Optimal. Sorting by optimal combines the three criteria to show the best available packages first.
Three essential JavaScript libraries
Thousands of JavaScript libraries have been written. Some have become so widely used that they’re considered essential. Essential JavaScript libraries sometimes become so essential that they become largely obsolete as the functionality they provided becomes part of the JavaScript language or browser APIs (as in the case of jQuery).
Any front-end developer should have at least a familiarity with what these essential libraries do so they’ll recognize them and can know when to use them.
This article introduces you to three of the most essential JavaScript libraries: Underscore, Lodash, and Moment.js. These libraries provide useful functions to solve problems programmers may encounter.
By knowing where to look for utility functions, you can save yourself the frustration and time involved in reinventing the wheel and end up with better code in the end.
Underscore.js
Underscore.js (https://underscorejs.org) provides more than 100 utility functions for working with arrays, collections, and objects in JavaScript. Underscore.js gets its name from the character that’s used to access its utilities: _.
Since Underscore’s creation in 2009, many of the functions that made it so useful have been, at least in part, incorporated into JavaScript. For example, the _.map() function creates a new array by applying a transformation function to each element of a list.
The built-in JavaScript Array.map() method can be used to accomplish the same thing as _.map() in most cases today. Other Underscore functions that have equivalents in the core JavaScript language include _.reduce(), _.find(), _.filter(), and _.each().
Lodash
Lodash (http://lodash.com) provides over 200 utility functions for simplifying common JavaScript tasks using a functional programming approach. Lodash got its start as a fork of Underscore.js, and, like Underscore you can use the _ symbol to access its functions.
Lodash provides implementations of many of the same utilities for working with Objects and Arrays as Underscore, plus many more.
Moment.js
Moment.js (https://momentjs.com) provides functions for formatting and working with dates in JavaScript. Some of its functions include
- moment.format(). Formats a date using a formatting string.
- moment.fromNow(). Calculates the difference between the current date and another date.
- moment.add() and moment.subtract(). These two functions are useful for adding or subtracting from a date.