Let’s begin the absolute positioning adventure by considering the default layout mechanism. Here, you can see an HTML5 page with two paragraphs on it. You can use CSS to give each paragraph a different color and to set a specific height and width. The positioning is left to the default layout manager, which positions the second (black) paragraph directly below the first (blue) one.
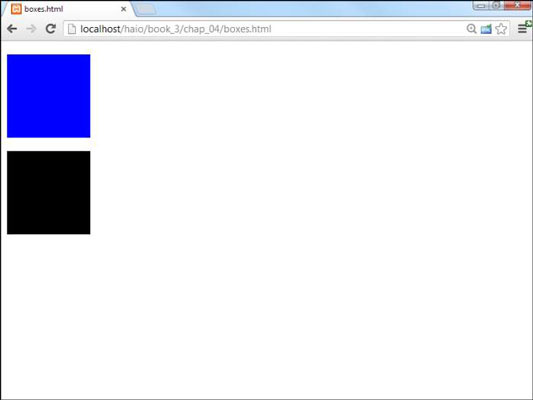
How to set up the HTML
The code is unsurprising:
<!DOCTYPE html> <html lang = "en-US"> <head> <meta charset = "UTF-8"> <title>boxes.html</title> <style type = "text/css"> #blueBox { background-color: blue; width: 100px; height: 100px; } #blackBox { background-color: black; width: 100px; height: 100px; } </style> </head> <body> <p id = "blueBox"></p> <p id = "blackBox"></p> </body> </html>
If you provide no further guidance, paragraphs (like other block-level elements) tend to provide carriage returns before and after themselves, stacking on top of each other. The default layout techniques ensure that nothing ever overlaps.
How to add position guidelines
Check out something new: The paragraphs are overlapping!
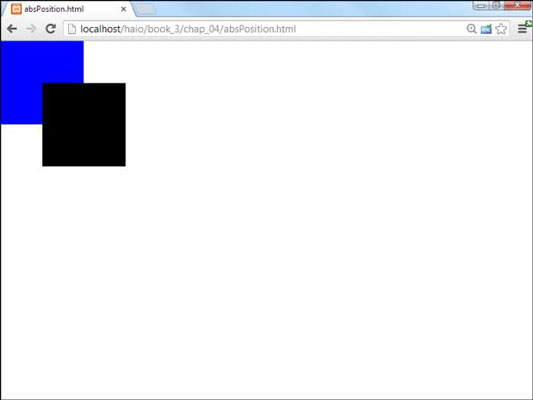
This feat is accomplished through some new CSS attributes:
<!DOCTYPE html> <html lang = "en-US"> <head> <meta charset = "UTF-8"> <title>absPosition.html</title> <style type = "text/css"> #blueBox { background-color: blue; width: 100px; height: 100px; position: absolute; left: 0px; top: 0px; margin: 0px; } #blackBox { background-color: black; width: 100px; height: 100px; position: absolute; left: 50px; top: 50px; margin: 0px; } </style> </head> <body> <p id = "blueBox"></p> <p id = "blackBox"></p> </body> </html>
So, why do you care if the boxes overlap? Well, you might not care, but the interesting part is this: You can have much more precise control over where elements live and what size they are. You can even override the browser's normal tendency to keep elements from overlapping, which gives you some interesting options.
How to make absolute positioning work
A few new parts of CSS allow this more direct control of the size and position of these elements. Here's the CSS for one of the boxes:
#blueBox { background-color: blue; width: 100px; height: 100px; position: absolute; left: 0px; top: 0px; margin: 0px; }
Set the position attribute to absolute.
Absolute positioning can be used to determine exactly (more or less) where the element will be placed on the screen:
position: absolute;
Specify a left position in the CSS.
After you determine that an element will have absolute position, it's removed from the normal flow, so you're obligated to fix its position. The left attribute determines where the left edge of the element will go. This can be specified with any of the measurement units, but it's typically measured in pixels:
left: 0px;
Specify a top position with CSS.
The attribute indicates where the top of the element will go. Again, this is usually specified in pixels:
top: 0px;
Use the height and width attributes to determine the size.
Normally, when you specify a position, you also want to determine the size:
width: 100px; height: 100px;
Set the margins to 0.
When you're using absolute positioning, you're exercising quite a bit of control. Because browsers don't treat margins identically, you're better off setting margins to 0 and controlling the spacing between elements manually:
margin: 0px;
Generally, you use absolute positioning only on named elements, rather than classes or general element types. For example, you won't want all the paragraphs on a page to have the same size and position, or you couldn't see them all. Absolute positioning works on only one element at a time.