This basic mechanism for storing data in PHP is great for small amounts of data, but it will quickly become unwieldy for HTML5 and CSS3 programming if you're working with a lot of information. If you're expecting hundreds or thousands of people to read your forms, you'll need a more organized way to store the data.
You can store data in a very basic text format that can be easily read by spreadsheets and databases. This has the advantage of imposing some structure on the data and is still very easy to manage.
The basic plan is to format the data in a way that it can be read back into variables. Generally, you store all of the data for one form on a single line, and you separate the values on that line with a delimiter, which is simply some character intended to separate data points.
Spreadsheets have used this format for many years as a basic way to transport data. In the spreadsheet world, this type of file is called a CSV (for comma-separated values) file. However, the delimiter doesn't need to be a comma. It can be nearly any character.
How to store data in a CSV file
Here's how you store data in a CSV file:
You can use the same HTML form.
The data is gathered in the same way regardless of the storage mechanism. There is a new page called addContactCSV.html, but the only difference between this file and the addContact.html page is the property. You can have the two pages send the data to different PHP programs, but everything else is the same.
Read the data as normal.
In your PHP program, you begin by pulling data from the previous form.
$lName = filter_input(INPUT_POST, "lName"); $fName = filter_input(INPUT_POST, "fName"); $email = filter_input(INPUT_POST, "email"); $phone = filter_input(INPUT_POST, "phone");
Store all the data in a single tab-separated line.
Concatenate a large string containing all the data from the form. Place a delimiter (like the tab symbol t) between variables and a newline (n) at the end.
//generate output for text file $output = $fName . "t"; $output .= $lName . "t"; $output .= $email . "t"; $output .= $phone . "n";
Open a file in append mode.
This time, the file is named contacts.csv to help remind the user that the contact form is now stored in a CSV format.
Write the data to the file.
The fwrite() function does this job with ease.
Close the file.
This part (like most of the program) is identical to the earlier version of the code.
Here's the code for addContactCSV.php in its entirety:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>addContactCSV.php</title> <link rel = "stylesheet" type = "text/css" href = "contact.css" /> </head> <body> <?php //read data from form $lName = filter_input(INPUT_POST, "lName"); $fName = filter_input(INPUT_POST, "fName"); $email = filter_input(INPUT_POST, "email"); $phone = filter_input(INPUT_POST, "phone"); //print form results to user print <<< HERE <h1>Thanks!</h1> <p> Your spam will be arriving shortly. </p> <p> first name: $fName <br /> last name: $lName <br /> email: $email <br /> phone: $phone </p> HERE; //generate output for text file $output = $fName . "t"; $output .= $lName . "t"; $output .= $email . "t"; $output .= $phone . "n"; //open file for output $fp = fopen("contacts.csv", "a"); //write to the file fwrite($fp, $output); fclose($fp); ?> </body> </html>
As you can see, this is not a terribly difficult way to store data.
How to view CSV data directly
This is what the resulting file looks like in a plain text editor.
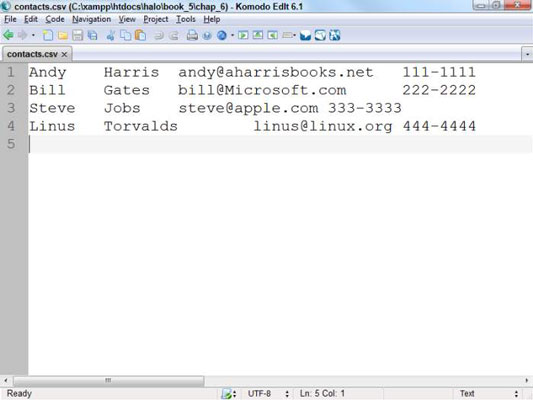
Of course, CSV data isn't meant to be read as plain text. On most operating systems, the .csv file extension is automatically linked to the default spreadsheet program. If you double-click the file, it will likely open in your spreadsheet.
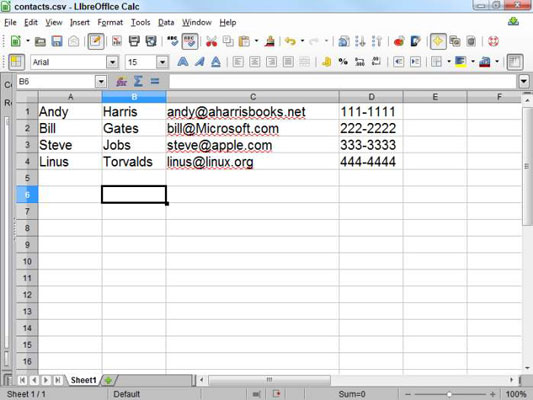
This is an easy way to store large amounts of data because you can use the spreadsheet to manipulate the data. Of course, relational databases are even better, but this is a very easy approach for relatively simple data sets.