setcookie()
function to set new cookies and update existing cookies. Here’s the basic format of the setcookie()
function:
>setcookie(<em>name</em> [, <em>value</em>] [, <em>expire</em>] [, <em>path</em>] [, <em>domain</em>] [, <em>secure</em>] [, <em>httponly</em>])The only required parameter is the name of the cookie, although you'll almost always want to include a cookie value, too. Leaving off the value sets the cookie value to
NULL
.The optional expire parameter allows you to specify the expiration date and time as a Unix timestamp value, making it a persistent cookie. The Unix timestamp format is an integer value of the number of seconds since midnight on January 1, 1970. The last four parameters allow you to specify the URL paths and domains allowed to access the cookie, and whether the cookie should be set as Secure
or HttpOnly
.
Be careful with the expire parameter. Even though the HTTP message sends the expire attribute as a full date and time, with the setcookie()
function you set it using a timestamp value, not a standard date and time. The way most PHP developers do that is by adding the number of seconds to the current date and time retrieved from the time()
function:
setcookie("test", "Testing", time() + (60*60*24*10));This sets the cookie named
test
to expire ten days from the time the web page is accessed by the site visitor.
Because the cookie is part of the HTTP message and not part of the HTML data, you must set the cookie before you send any HTML content, including the opening tag. There is an exception to this, though. If the PHP output_buffer
setting is enabled, the PHP server sends all output from the program to a buffer first. Then, either when the buffer is full or the program ends, it rearranges the data in the buffer to place the HTTP messages first and then sends the data to the client browser.
- Open your favorite text editor, program editor, or integrated development environment (IDE) package.
- Type the following code into the editor window:
<?php setcookie("test1", "This is a test cookie", time() + 600); ?> <!DOCTYPE html> <html> <head> <title>PHP Cookie Test</title> </head> <body> <h1>Trying to set a cookie</h1> </body> </html>
- Save the file as
cookietest1.php
in theDocumentRoot
folder for the web server.For XAMPP in Windows, that'sc:\xampp\htdocs
; for XAMPP in macOS, that’s/Applications/XAMPP/htdocs
. - Start the XAMPP Control Panel and then start the Apache web server.
- Test your code here.You may need to change the TCP port number to match your web server.
- Using your browser's Developer Tools, check the cookies that are set from the web page and their expiration date and time. You should see the
test1
cookie created.It should be set to expire in ten minutes. - Close the browser window when you’re done.
test1
cookie that was set by the program. For the Microsoft Edge browser, look in the Debugger section for the cookies.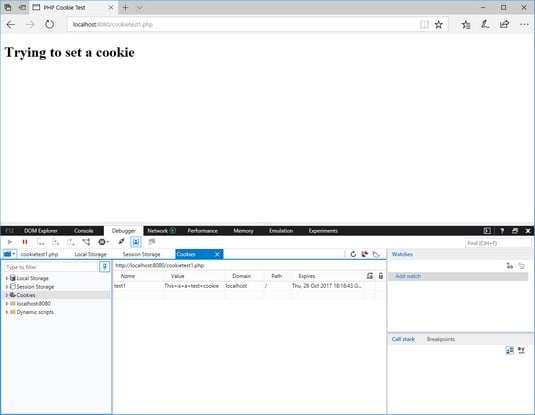
The cookie is set, along with the value, and the expiration time is set to ten minutes (600 seconds) in the future.
You have to place the setcookie()
function lines before the html
section of the web page. Otherwise, you'll get an error message. The web server must send any cookie data in the HTTP session before any HTML content.