Most applications require some level of configuration. The user or administrator selects application options or the application itself detects environmental needs. The kinds of input for configuration vary, but the fact remains that applications need to know how to interact with the user in a meaningful way through settings that the application reads each time it starts. The settings are normally stored in a configuration file on disk. Depending on the application, you can use any of a number of storage techniques, but some applications can get by with a simple text file. In fact, that’s what the example application does — it uses a simple text file to store configuration information.
This example makes use of a number of standard C++ programming techniques, and yet all it really does is read and write a file in a specific way. Here’s the code you need for this application.
#include <iostream> #include <fstream> #include <string> using namespace std; int main() { ifstream cfile("Config.config"); string Name; string Greeting; if (cfile.good()) { cfile >> Name; cfile >> Greeting; cout << Greeting << " " << Name << endl; } else { cfile.close(); ofstream cfile("Config.config"); cout << "What is your name? " << endl; cin >> Name; cout << "How do you want to be greeted?" << endl; cin >> Greeting; cfile << Name << endl; cfile << Greeting << endl; } cfile.close(); return 0; }
The example begins by creating an ifstream object, cfile, that points to Config.config on the hard drive. If the file exists, then cfile.good() returns true and the application can read the settings from the file. It then displays the greeting and the name something like what you see below.

However, if this is the first time that the application has been run, then Config.config won’t exist on the hard drive. In this case, the application closes the ifstream object and creates a new ofstream object. It then asks the user to provide a username and greeting as shown here:
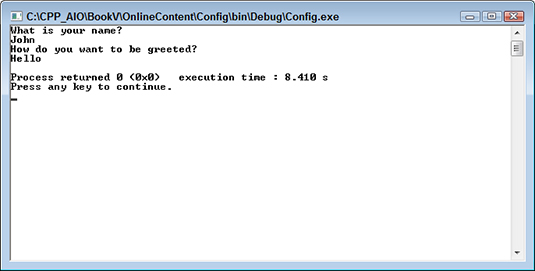
These settings are then stored in Config.config for later use. Each time the application is run, the user sees the specified greeting.
The most common problem developers have when creating this sort of application is remembering which way to point the arrows when working with a stream such as cin, cout, and cfile. The easiest way to overcome this problem is to always point the arrows in the direction of the container that will receive the data. If you remember this little trick, you’ll have fewer problems working with files.