Often, you'll want to do something in PHP as simple as record information from a form into a text file for HTML5 and CSS3 programming. Here is a simple program that responds to a form and passes the input to a text form.
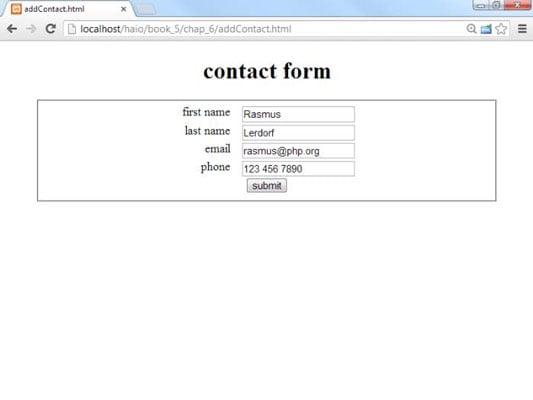
The code for this form is basic HTML.
When the user enters contact data into this form, it will be passed to a program that reads the data, prints out a response, and stores the information in a text file.
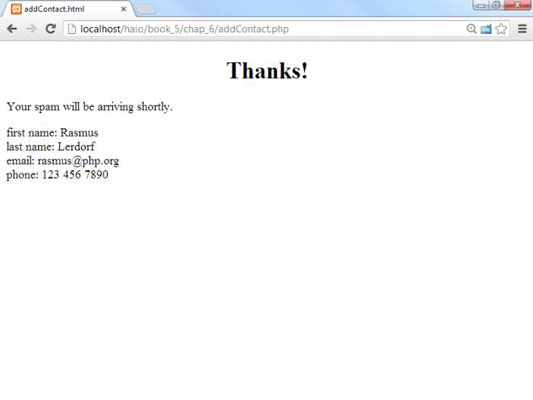
The more interesting behavior of the program is not visible to the user. The program opens a file for output and prints the contents of the form to the end of that file. Here are the contents of the data file after a few entries:
first: Andy last: Harris email: [email protected] phone: 111-1111 first: Bill last: Gates email: [email protected] phone: 222-2222 first: Steve last: Jobs email: [email protected] phone: 333-3333 first: Linus last: Torvalds email: [email protected] phone: 444-4444 first: Rasmus last: Lerdorf email: [email protected] phone: 123 456 7890
The program to handle this input is not complicated. It essentially grabs data from the form, opens up a data file for output, and appends that data to anything already in the file. Here's the code for addContact.php:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>addContact.html</title> <link rel = "stylesheet" type = "text/css" href = "contact.css" /> </head> <body> <?php //read data from form $lName = filter_input(INPUT_POST, "lName"); $fName = filter_input(INPUT_POST, "fName"); $email = filter_input(INPUT_POST, "email"); $phone = filter_input(INPUT_POST, "phone"); //print form results to user print <<< HERE <h1>Thanks!</h1> <p> Your spam will be arriving shortly. </p> <p> first name: $fName <br /> last name: $lName <br /> email: $email <br /> phone: $phone </p> HERE; //generate output for text file $output = <<< HERE first: $fName last: $lName email: $email phone: $phone HERE; //open file for output $fp = fopen("contacts.txt", "a"); //write to the file fwrite($fp, $output); fclose($fp); ?> </body> </html>
The process is straightforward:
Read data from the incoming form.
Just use the filter_input mechanism to read variables from the form.
Report what you're doing.
Let users know that something happened. As a minimum, report the contents of the data and tell them that their data has been saved. This is important because the file manipulation will be invisible to the user.
Create a variable for output.
In this simple example, you print nearly the same values to the text file that you reported to the user. The text file does not have HTML formatting because it's intended to be read with a plain text editor. (Of course, you could save HTML text, creating a basic HTML editor.)
Open the file in append mode.
You might have hundreds of entries. Using append mode ensures that each entry goes at the end of the file, rather than overwriting the previous contents.
Write the data to the file.
Using the fput() or fwrites() function writes the data to the file.
Close the file.
Don't forget to close the file with the fclose() function.
The file extension you use implies a lot about how the data is stored. If you store data in a file with an .txt extension, the user will assume it can be read by a plain text editor.
The .dat extension implies some kind of formatted data, and .csv implies comma-separated values. You can use any extension you want, but be aware you will confuse the user if you give a text file an extension like .pdf or .doc.