Object-oriented programming in PHP has another feature which makes it very useful for large projects. Many objects are related to each other, and you can use a family tree relationship to simplify your programming.
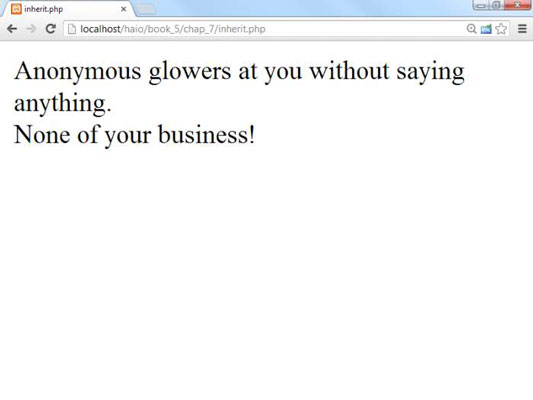
How to build a critter based on another critter
There's a new critter in town. This one has the same basic features, but a worse attitude. Take a look at the code to see what's going on:
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <title>inherit.php</title> </head> <body> <?php require_once("Critter.php"); class BitterCritter extends Critter{ //all properties and methods inherited from Critter //You can add new properties and methods public function glower(){ return "$this->name glowers at you without saying anything."; } // end glower //if you over-write an existing method, the behavior changes public function talk(){ return "None of your business!"; } // end talk } // end class def $a = new BitterCritter(); print $a->glower() . "<br />"; print $a->talk() . "<br />"; ?> </body> </html>
This example is an illustration of a very common programming situation, where you want a specialization of a previously defined class. There is already have a Critter class, but you want a new kind of Critter. The new critter (the BitterCritter) begins with the same general characteristics of the ordinary critter, but brings a new twist. The object-oriented idea of inheritance is a perfect way to handle this situation.
JavaScript supports a different form of object-oriented programming based on an idea called prototyping rather than inheritance. People have long and boring conversations about which technique is better, but ultimately it doesn't matter much. Most OOP languages support the form of inheritance used in PHP, so you should really know how it works.
How to inherit the wind (and anything else)
Here's how to implement inheritance:
Begin with an existing class.
For this example, you begin with the ordinary Critter class, which you import with the require_once() function.
Create your new class with the extends keyword.
As you define the class, if you use the extends keyword to indicate which class you are inheriting, your new class will begin with all the properties and methods of the parent class.
You can access public and protected elements of the parent, but not private ones.
If a property or method was defined as private in the original class, it's truly nobody else's business. No other code fragments can access that element. Generally though, when you inherit from a class, the new child class should have access to the parent class's elements. That's why you should create properties as protected rather than private.
Add new properties and methods.
You can extend your new class with additional properties and methods that the parent did not have. The BitterCritter now features a glower() method that ordinary critters do not have.
You can also overwrite parent behavior.
If you redefine a method that the parent class had, you are changing the behavior of the new class. This allows you to modify existing behaviors (a form of an object-oriented idea called polymorphism).
This demonstration is just the barest glimpse into object-oriented programming. There is much more to this form of software development, but the basics are all here. Though you might not immediately see the need to build your own objects from scratch, you will definitely encounter object-oriented PHP code as you begin exploring more complex ideas like data programming and content management systems.