If you can write data to a file in PHP, it would make sense that you could read from that file for HTML5 and CSS3 programming as well. The readContact.php program pulls the data saved in the previous program and displays it to the screen.
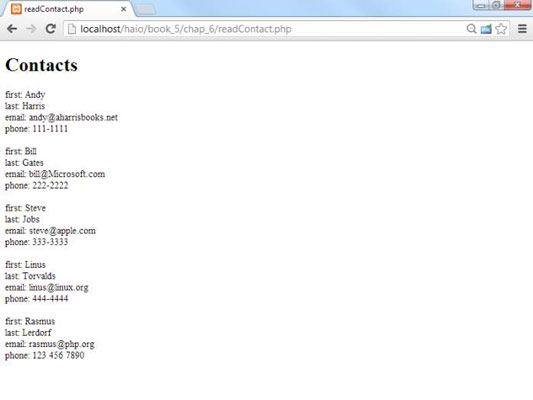
It is not difficult to write a program to read a text file. Here's the code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>readContact.php</title> </head> <body> <h1>Contacts</h1> <div> <?php //open up the contact file $fp = fopen("contacts.txt", "r") or die("error"); //print a line at a time while (!feof($fp)){ $line = fgets($fp); print "$line <br />"; } //close the file fclose($fp); ?> </div> </body> </html>
The procedure is similar to writing the file, but it uses a while loop.
Open the file in read mode.
Open the file just as you do when you write to it, but use the designator to open the file for read mode. Now you can use the fgets() function on the file.
Create a while loop for reading the data.
Typically, you'll read a file one line at a time. You'll create a while loop to control the action.
Check for the end of the file with.
You want the loop to continue as long as there are more lines in the file. The feof() function returns the value if you are at the end of the file and false if there are more lines to read. You want to continue as long as feof()returns false.
The exclamation point (!) operator is a logical not. The condition !feof($fp) is true when there is data left in the file and false when there are no lines left, so this is the appropriate condition to use here.
Read the next line with the fgets() function.
This function reads the next line from the file and passes that line into a variable (in this case, $line).
Print out the line.
With the contents of the current line in a variable, you can do whatever you want with it. You could format the contents, search for a particular value, or whatever else you want.