Functions in programming are a simple way to group together actions. With them you can do some groups of actions repeatedly without having to retype all the code. You save typing, and it's easier to think about how to structure your program and to update it.
One problem with these approaches: They don't let you reuse code in other circumstances. Besides comments, which get messy as programs get larger, you have no obvious way to tell what part of the code does what.
Think of when your parents tell you to get ready for school. They might say, "Get up, get dressed, have some breakfast, put your homework in your bag, put your lunch in your bag, brush your teeth." When they say, "Get ready for school," they're wrapping all those separate activities into one thing, like a get_ready function. They're also moving from the particular (detailed instructions on what to do and how to do it) to the general (getting ready). This is called abstraction.
Whenever you move from thinking less about details, you're being more abstract (or you've accidentally gone to sleep). When you abstract things, you can plan with general concepts but tackle each separate task. Plan your own programs using functions as your level of abstraction, then tackle what each function does separately. Divide, then conquer!
Functions let you explain what does what, and functions let you reuse your code.
To use a function, you must
Define the function itself
Invoke, or call, the function
Here's a simple example that reworks your Hello World! program using a function. Open IDLE and type this in the Shell window:
>>> def print_hello_world(): ""Hello World as a function"" print('Hello World!')
Remember to press Enter twice to return to the command prompt.
It doesn't do anything. A bit pointless? Sort of. You're defining the function (using the def keyword). Now, you must call the function to make it run:
>>> print_hello_world() Hello World!
As you can see from Figure 1, Python will call the function if you write the function's name followed by parentheses. This means that as Python flows through your program, when it reaches the function call it continues at the function definition and runs through the code in that function's code block.
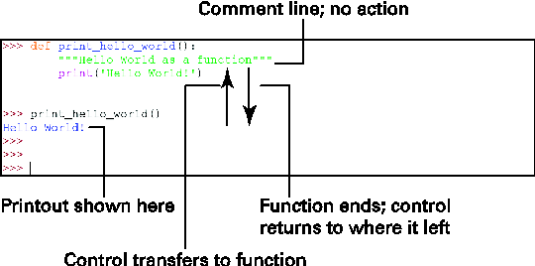
A function's code block is the line following the def statement up to (but not including) the next line that's indented the same as the def statement. You can see some code blocks pointed out in Figure 2.
When the Python reaches the end of the function, it skips back to the spot where the call occurred (back there with the parentheses).
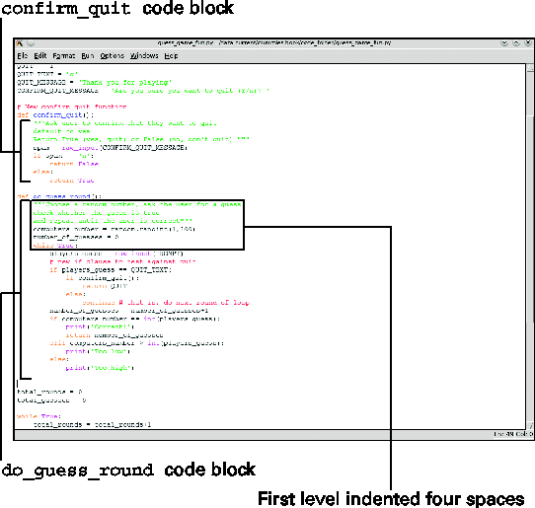
Including the parentheses with the function call is important. If you leave them out Python doesn't call the function. Instead it tells you about the function:
>>> print_hello_world <function print_hello_world at 0x7f8dd8043b90>
Here it's telling you that print_hello_world is a function, and that its name is print_hello_world. The bit at the end is where in memory the Python keeps the function.