A few primitive shapes can be drawn directly onto the graphics context in JavaScript. The most common shapes for HTML5 and CSS3 programming that most web developers use are rectangles and text.
Rectangle functions
You can draw three different types of rectangles:
clearRect(x, y, w, h): Erases a rectangle with the upper-left corner (x,y) and size (w,h). Generally, erasing draws in the background color.
fillRect(x, y, w, h): Draws a box with upper-left corner (x, y) and size (w,h). The rectangle is filled in with the currently-defined fillStyle.
strokeRect(x, y, w, h): Draws a box with upper-left corner (x, y) and size (w,h). The box is not filled in, but the outline is drawn in the currently-defined strokeStyle and using the current lineWidth.
Here's the code:
function draw(){ //from rectangle.html var drawing = document.getElementById("drawing"); var con = drawing.getContext("2d"); con.fillStyle = "red"; con.strokeStyle = "green"; con.lineWidth = "5"; con.fillRect(10, 10, 180, 80); con.strokeRect(10, 100, 180, 80); } // end draw
How to draw text
The tag has complete support for text. You can add text anywhere on the canvas, using whichever font style and size you wish.

Text is drawn onto canvas much like a rectangle. The first step is to pick the desired font. Canvas fonts are created by assigning a font to the context's font attribute. Fonts are defined like the single-string font assignment in CSS. You can specify all of the font characteristics in the same order you do when using the font shortcut: style, variant, weight, size, and family.
When you're ready to display actual text on the screen, use the fillText() method, which accepts three parameters. The first parameter is the text to display. The last two parameters are the X and Y position of the left-hand side of the text. The following code is used to produce the result. When the strokeStyle is not explicitly set, the stroke is black by default.
function draw(){ //from text.html var drawing = document.getElementById("drawing"); var con = drawing.getContext("2d"); //clear background con.fillStyle = "white"; con.fillRect(0,0, 200, 200); // draw font in red con.fillStyle = "red"; con.font = "20pt sans-serif"; con.fillText("Canvas Rocks!", 5, 100); con.strokeText("Canvas Rocks!", 5, 130); } // end draw
How to add shadows
You can add shadows to anything you draw on the canvas. Shadows are quite easy to build. They require a number of methods of the context object:
shadowOffsetX: Determines how much the shadow will be moved along the X axis. Normally this will be a value between 0 and 5. A positive value moves the shadow to the right of an object. Change this value and the shadowOffsetY value to alter where the light source appears to be.
shadowOffsetY: Determines how far the shadow is moved along the X axis. A positive value moves the shadow below the object. In general, all shadows on a page should have the same X and Y offsets to indicate consistent lighting. The size of the offset values implies how high the element is "lifted" off the page.
shadowColor: The shadow color indicates the color of the shadow. Normally this is defined as black, but the color can be changed to other values if you wish.
shadowBlur: The shadowBlur effect determines how much the shadow is softened. If this is set to 0, the shadow is extremely crisp and sharp. A value of 5 leads to a much softer shadow. Shadow blur generally lightens the shadow color.
If you apply a shadow to text, be sure that the text is still readable. Large simple fonts are preferred, and you may need to adjust the shadow color or blur to ensure the main text is still readable. After you've applied shadow characteristics, all subsequent drawing commands will incorporate the shadow. If you want to turn shadows off, set the shadowColor to a transparent color using RGBA.
Here's the code to produce text with a shadow:
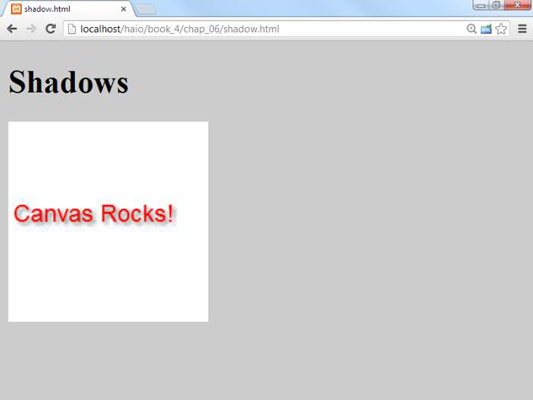
<!DOCTYPE HTML> <html lang = "en"> <head> <title>shadow.html</title> <meta charset = "UTF-8" /> <style type = "text/css"> body { background-color: #cccccc; } </style> <script type = "text/javascript"> function draw(){ //from shadow.html var drawing = document.getElementById("drawing"); var con = drawing.getContext("2d"); //clear background con.fillStyle = "white"; con.fillRect(0,0, 200, 200); // draw font in red con.fillStyle = "red"; con.font = "18pt sans-serif"; //add shadows con.shadowOffsetX = 3; con.shadowOffsetY = 3; con.shadowColor = "gray"; con.shadowBlur = 5; con.fillText("Canvas Rocks!", 5, 100); } // end draw </script> </head> <body onload = "draw()"> <h1>Shadows</h1> <canvas id = "drawing" height = "200" width = "200"> <p>Canvas not supported!</p> </canvas> </body> </html>