Real-life HTML5 and CSS3 programming is dangerous. Lots of things can go wrong in PHP. So the smart way to program data is defensive programming. This practice involves anticipating errors and trying to resolve them gracefully. PHP has some advanced error-handling techniques available which are perfect for the task.
Imagine you wrote some code that looked like this:
print 5 / 0;
You probably wouldn't do that, but sometimes bad code slips through. If your server is set up to pass out error messages, you'll see something like this.
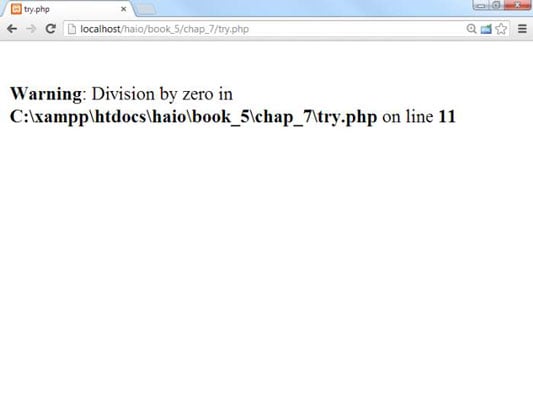
Exception handling
There's actually a lot more going on than you might appreciate at first. The default behavior of many PHP installations is to hide errors. However, errors occur, especially if you allow user input. This code listing explicitly traps for errors and reports them regardless of server settings:
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <title>try.php</title> </head> <body> <p> <?php try { 5 / 0; } catch (Exception $e){ print $e->getMessage(); } // end try ?> </p> </body> </html>
Here's what's happening:
Use the try keyword to indicate potentially dangerous code.
The try keyword opens up a block of code (like a loop or condition). All the code between try and catch is considered potentially dangerous.
Place dangerous code in the try block.
Any code that might cause errors should be placed inside the try block. The most dangerous code usually involves things the programmer can't directly control: access to external files, operations on user-defined data, or exposure to external programs and processes.
Use the catch clause to anticipate errors.
The catch clause indicates the end of the dangerous code.
Indicate the exception type.
The parameter for the catch clause is an object of type Exception. PHP has a number of built-in exceptions, and often a library or toolset will include new exceptions (you can also build them yourself if you want).
Manage the exception.
The catch clause opens another block of code. Put the code in here that will resolve the problem (or at least die with a little style and grace — informing the user what went wrong before shuffling off this mortal coil). The most common line here is to call print($e->getMessage()). All exception objects have a getMessage() method, and this line reports the current error message.
Knowing when to trap for exceptions
If your server is set up for debugging (as XAMPP is by default), it won't usually be necessary to set up exception handling because the default behavior of a debug setup is to report the exceptions anyway. There are a few times you'll still want explicit exception handling:
You're on a server without debug settings: You may not have access to the server configuration, so you might not be able to turn on automatic exception reports. Manual exception reports still get through.
You want to do something special: The automatic exception handler simply reports the problem. If you want to do something else (say, use a default file if a file is not found), you'll need a custom exception handler for that situation.
You're doing something exotic: Special libraries often come with their own custom exceptions, and you'll need an exception handler to cover these situations.