An important technique in HTML5 and CSS3 web development is the use of a tabbed interface in AJAX. This allows the user to change the contents of a segment by selecting one of a series of tabs.
In a tabbed interface, only one element is visible at a time, but the tabs are all visible. The tabbed interface is a little more predictable than the accordion because the tabs (unlike the accordion's headings) stay in the same place.
The tabs change colors to indicate which tab is currently highlighted, and they also change state (normally by changing color) to indicate that they are being hovered over. When you click another tab, the main content area of the widget is replaced with the corresponding content.
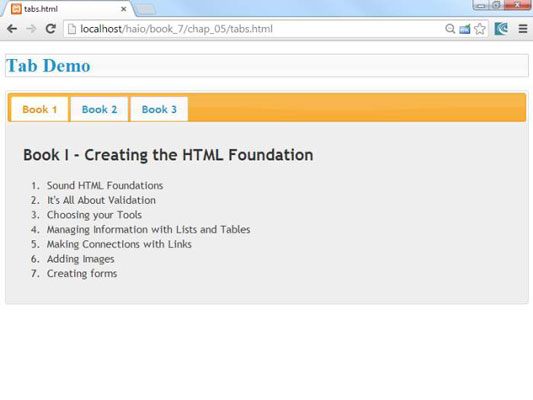
This is what happens when the user clicks the tab.
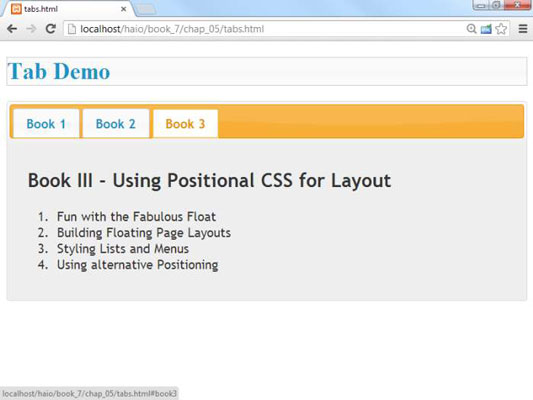
Like the accordion, the tab effect is incredibly easy to achieve. Look over the code:
<!DOCTYPE html> <html lang = "en-US"> <head> <meta charset = "UTF-8" /> <link rel = "stylesheet" type = "text/css" href = "css/ui-lightness/jquery-ui-1.10.3.custom.css" /> <script type = "text/javascript" src = "js/jquery-1.9.1.js"></script> <script type = "text/javascript" src = "js/jquery-ui-1.10.3.custom.min.js"></script> <script type = "text/javascript"> $(init); function init(){ $("#tabs").tabs(); } </script> <title>tabs.html</title> </head> <body> <h1 class = "ui-state-default">Tab Demo</h1> <div id = "tabs"> <ul> <li><a href = "#book1">Book 1</a></li> <li><a href = "#book2">Book 2</a></li> <li><a href = "#book3">Book 3</a></li> </ul> <div id = "book1"> <h2 id="tab1" >Book I - Creating the HTML Foundation</h2> <ol> <li>Sound HTML Foundations</li> <li>It's All About Validation</li> <li>Choosing your Tools</li> <li>Managing Information with Lists and Tables</li> <li>Making Connections with Links</li> <li>Adding Images</li> <li>Creating forms</li> </ol> </div> <div id = "book2"> <h2 id="tab2" >Book II - Styling with CSS</h2> <ol> <li>Coloring Your World</li> <li>Styling Text</li> <li>Selectors, Class, and Style</li> <li>Borders and Backgrounds</li> <li>Levels of CSS</li> </ol> </div> <div id = "book3"> <h2 id="tab3" >Book III - Using Positional CSS for Layout</h2> <ol> <li>Fun with the Fabulous Float</li> <li>Building Floating Page Layouts</li> <li>Styling Lists and Menus</li> <li>Using alternative Positioning</li> </ol> </div> </div> </body> </html>
The mechanism for building a tab-based interface is very similar to the one for accordions:
Add all the appropriate files.
Like most jQuery UI effects, you need jQuery, jQuery UI, and a theme CSS file. You also need access to the images directory for the theme's background graphics.
Build HTML as normal.
If you're building a well-organized web page anyway, you're already pretty close.
Build a div that contains all the tabbed data.
This is the element that you'll be doing the jQuery magic on.
Place main content areas in named divs.
Each piece of content that will be displayed as a page should be placed in a div with a descriptive ID. Each of these divs should be placed in the tab div.
Add a list of local links to the content.
Build a menu of links. Place this at the top of the tabbed div. Each link should be a local link to one of the divs. For example, the index looks like this:
<ul> <li><a href = "#book1">Book 1</a></li> <li><a href = "#book2">Book 2</a></li> <li><a href = "#book3">Book 3</a></li> </ul>
Build an init() function as usual.
Use the normal jQuery techniques.
Call the tabs() method on the main div.
Incredibly, one line of jQuery code does all the work.