Because C++ is so incredibly flexible, people keep coming to it as a best solution for many general programming needs and some specific needs as well.
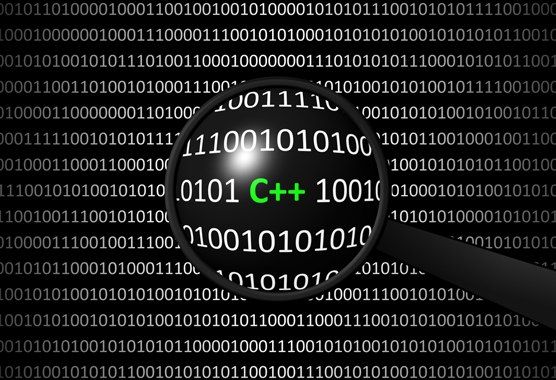
C++ Programming Paradigms
You can program in C++ in more than one way. Most people think you’re limited to using just procedural or object-oriented programming techniques. However, if you stop there, you’re missing out on half the fun of working with C++. The following list describes the various C++ programming paradigms in common use today:
- Imperative: Imperative programming takes a step-by-step approach to performing a task. The developer provides commands that describe precisely how to perform the task from beginning to end. During the process of executing the commands, the code also modifies application state, which includes the application data. The code runs from beginning to end. An imperative application closely mimics the computer hardware, which executes machine code. Machine code is the lowest set of instructions that you can create and is mimicked in early languages, such as assembler.
- Procedural: Procedural programming implements imperative programming, but adds functionality such as code blocks and procedures for breaking up the code. The compiler or interpreter still ends up producing machine code that runs step by step, but the use of procedures makes it easier for a developer to follow the code and understand how it works. Many procedural languages provide a disassembly mode in which you can see the correspondence between the higher-level language and the underlying assembler.
- Object-oriented: The procedural paradigm does make reading code easier. However, the relationship between the code and the underlying hardware still makes it hard to relate what the code is doing to the real world. The object-oriented paradigm uses the concept of objects to hide the code, but more important, to make modeling the real world easier. A developer creates code objects that mimic the real-world objects they emulate. These objects include properties, methods, and events to allow the object to behave in a particular manner.
- Functional: Functional programming has somewhat different goals and approaches than other paradigms use. Goals define what the functional programming paradigm is trying to do in forging the approaches used by languages that support it. However, the goals don’t specify a particular implementation; doing that is within the purview of the individual languages. The main difference between the functional programming paradigm and other paradigms is that functional programs use math functions rather than statements to express ideas. This difference means that rather than write a precise set of steps to solve a problem, you use math functions, and you don’t worry about how the language performs the task.
- Declarative: Functional programming actually implements the declarative programming paradigm, but the two paradigms are separate. Other paradigms, such as logic programming, implemented by the Prolog language, also support the declarative programming paradigm. The short view of declarative programming is that it does the following: describes what the code should do, rather than how to do it; defines functions that are referentially transparent (without side effects); and provides a clear correspondence to mathematical logic.
10 Common C++ Mistakes
It’s possible to make mistakes in coding, even if you’re not aware of them. A lack of knowledge about a new programming requirement is still a mistake, but one that you might have a very hard time finding. Although many C++ programmers take measures to prevent bugs, mistakes still slip through. This list of ten common mistakes while writing C++ code can help both new and veteran programmers:
- Failure to declare the variable
- Using the wrong uppercase and lowercase letters; for example, you typed Main when you meant main
- Employing one equals sign (
=
) when you were supposed to use two (==
), either in an if statement or in a for - Leaving out
#include <iostream>
or usingnamespace std;
- Relying on older programming techniques, language features, or library calls after upgrading to a newer version of C++
- Forgetting to call
new
and using the pointer anyway - Omitting the word
public:
in your classes, so everything turned up private - Creating ambiguous references to classes when working with multiple libraries so that your code ends up using the wrong library
- Leaving off the parentheses when calling a function that takes no parameters
- Skipping a semicolon, probably at the end of a class declaration, or other necessary punctuation
Overview of the Standard Library Categories
The Standard Library is immense and becoming larger all the time. No one can possibly master such a large coding option, yet developers still have to find their way around it. The best way to begin is to break the Standard Library into smaller pieces. You can categorize the Standard Library functions in a number of ways. One of the most common approaches is to use the following categories:
- Algorithms: Performs data manipulations such as replacing, locating, or sorting information
- Atomic Operations (C++ 11 and above): Allows construction of a code block that executes as a single concurrent entity without the use of locking mechanisms
- C Compatibility: Provides you with access to functionality that came with the original C language
- Concepts (C++ 20 and above): Provides predicates that express a generic algorithm’s expectations through concepts; You use a concept to formally document the constraints on a template to enforce certain behaviors.
- Containers: Allows storage of various kinds of data using a specific approach; For example, both queues and deques are kinds of containers.
- Coroutines (C++ 20 and above): Allows a function to suspend execution and resume its task later
- Filesystem (C++ 17 and above): Provides functionality needed to work with file systems on a local system
- Input/Output: Makes it possible to access various forms of I/O, such as files, the console, and network streams
- Iterators: Enumerates various kinds of data; Creates lists of items and manipulate them in specific ways.
- Localization: Performs these tasks:
- Character classification
- String collation
- Numeric, monetary, and date/time formatting and parsing
- Message retrieval
- Numerics: Provides access to all sorts of functions to perform math-related tasks
- Ranges (C++ 20 and above): Works with views, which describe what you want to see as output, to enumerate, manipulate, and otherwise manage ranges of data
- Regular Expressions (C++ 11 and above): Helps you look for patterns in strings
- Strings: Makes strings easier to use by allowing data conversions, formatting, and other sorts of string manipulations
- Thread Support (C++ 11 and above): Allows parallel and threaded execution of tasks
- Utilities: Gives access to functions and types that perform small service tasks within the Standard Library
Reasons to Use a Vector
Some people view vectors and arrays as essentially interchangeable. However, vectors are different from arrays, and you need to ensure that you know how to use them to code effectively. There are a number of advantages to using a vector instead of a regular, plain old, no-frills array:
- You don’t need to know up front how many items will be going in it. With an array, you need to know the size when you declare it.
- You don’t need to specifically deallocate a vector as you do a dynamically defined array.
- It’s possible to obtain the precise size of a vector, so you don’t need to pass the size of the vector to a function.
- When a vector is filled, the underlying code allocates additional memory automatically.
- You can return a vector from a function. To return an array, you must dynamically define it first.
- You can copy or assign a vector.